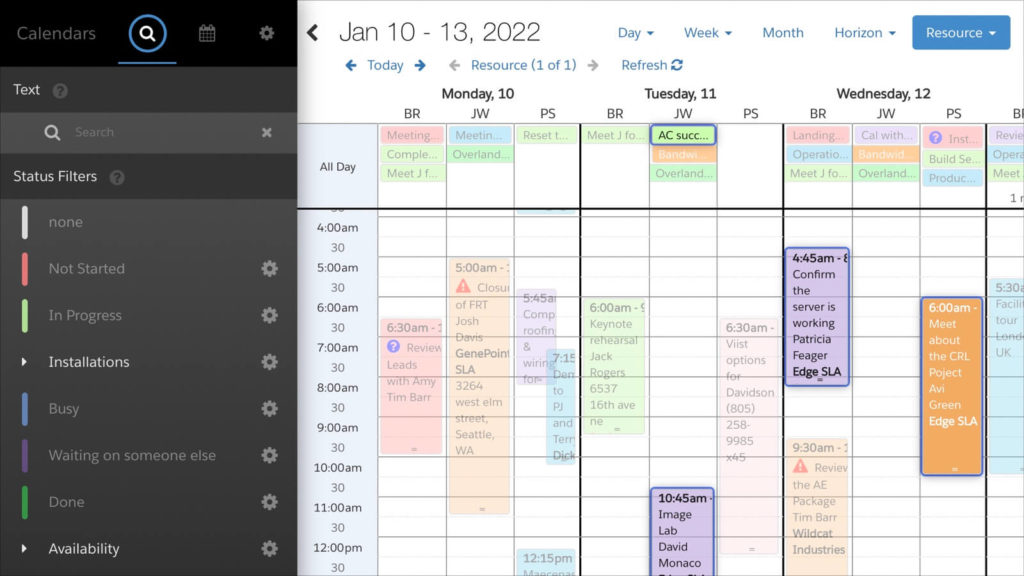
DayBack supports selecting multiple items on the calendar by shift-clicking on them, as documented here. However, what if we want to use custom logic to determine which items are selected.
For example, what if we want to quickly select items that share the same resource, contact, status, or another facet of the items we are viewing. How can we set that up?
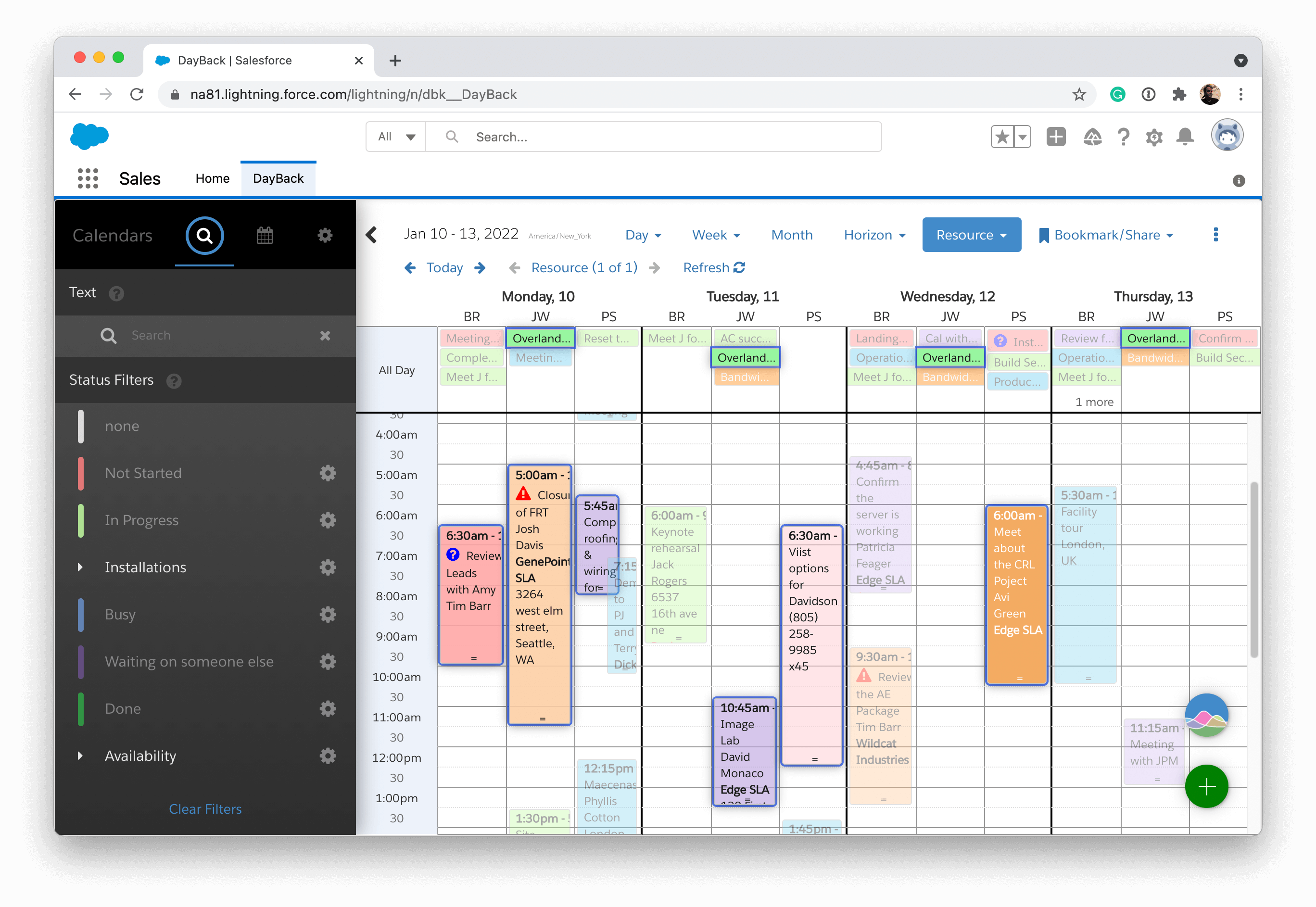
Capturing the On Click JavaScript Event
DayBack’s On Click event actions include the click event within their scope and can be referenced via the scope variable jsEvent. This gives developers the ability to get additional information about the click event when clicking on an event in the calendar. You can capture things like modifier keys and then branch the code within the On Click action based on which modifier key is being pressed at the time.
New to JavaScript?
We present these strategies and sample code as customizations you can undertake yourself, but we’re also available to make customizations for you as part of implementation packages for DayBack.
We can’t use the shift key for this, as that’s already dedicated for multi-selecting items one at a time, so in this example, the action will detect if the option key is being pressed when an item is clicked and then run the custom routine if it is. If the option key isn’t being pressed, the action will continue with its default behavior. Be sure to specify “Prevent Default Action” to Yes so that the action will not just open the event in the popover but run the custom routine instead.
If ( jsEvent and jsEvent.altkey ) { //the option key has been pressed, run a custom routine to multi-select events //define custom routine here … //stop the default behavior for the On Click Event cancelCallback(); } else { //option key is not pressed, let action run with default behavior confirmCallback(); }
Multi-Select Function is Available in the Custom Event Action Scope
The function DayBack uses to multi-select items is available in the scope of Custom Event Actions. So once we’ve determined that the appropriate modifier key is selected, we can then loop through all the items in the DOM and then multi-select any that match our criteria. The multi-select function follows:
toggleMultiSelect(event, shiftKey, targetElement, view, forceDeselect);
The arguments that we’re interested in for this example are the event and targetElement; the rest can just be hard coded. The event is the DayBack event object itself, and the targetElement is the associated DOM element. The targetElement is required so that its class can be updated to display that it has been selected. In our On Click action, we’ll include a function that will find the DOM element for a specific event:
function getElement(id){ var string = '[data-id=\'' + id + '\']'; var a = document.querySelectorAll(string); var result = a[0]; return result; }
Now, if we want to select all items that have the same opportunity as an item we’ve clicked, we can do the following:
var thisProject = event.projectName[0]; var clientEvents = seedcodeCalendar.get('element').fullCalendar('clientEvents'); var view = seedcodeCalendar.get('view'); for ( var i = 0 ; i < clientEvents.length ; i++){ if ( thisProject && ( clientEvents[i].projectName[0]===thisProject ) ){ //this event has the same project, add to multi-select var thisEvent = clientEvents[i]; var thisElement = getElement(clientEvents[i]._id); toggleMultiSelect(thisEvent,true,thisElement,view,false); } }
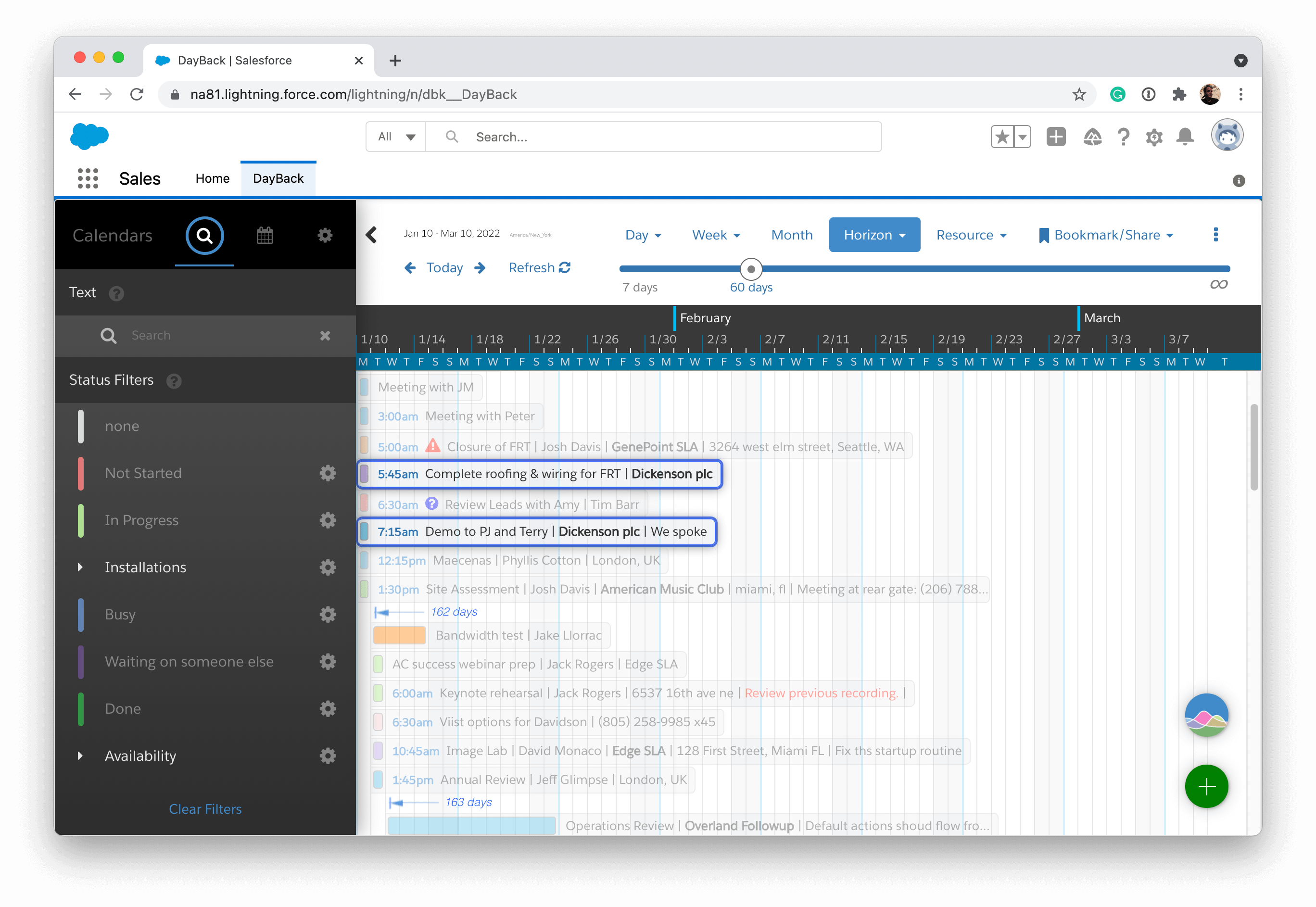
You can also use this technique in conjunction with DayBack’s text filter to find items for a specific project: option-click on one of them and then clear the text filter to see the items selected and in the context of the whole calendar.
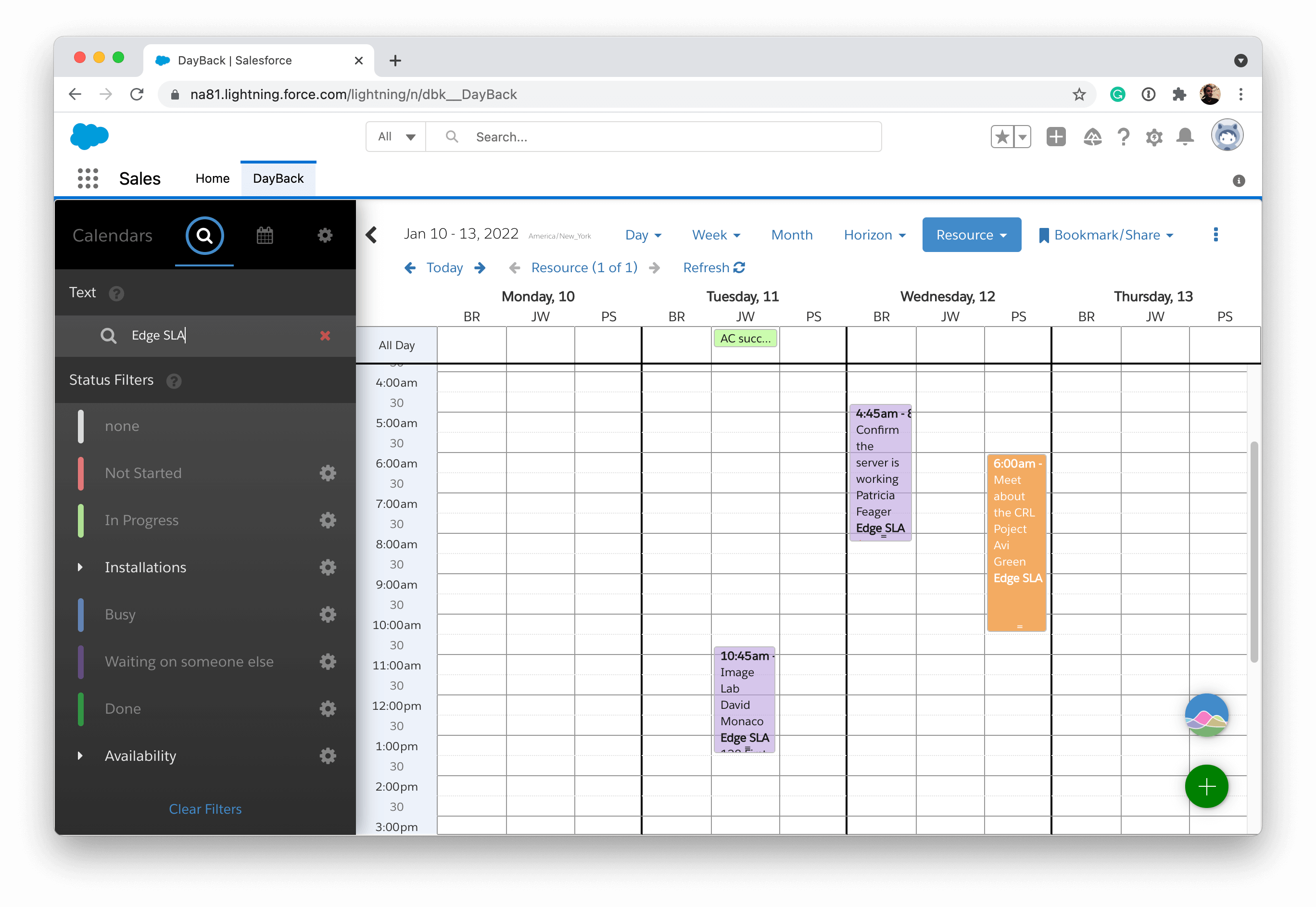
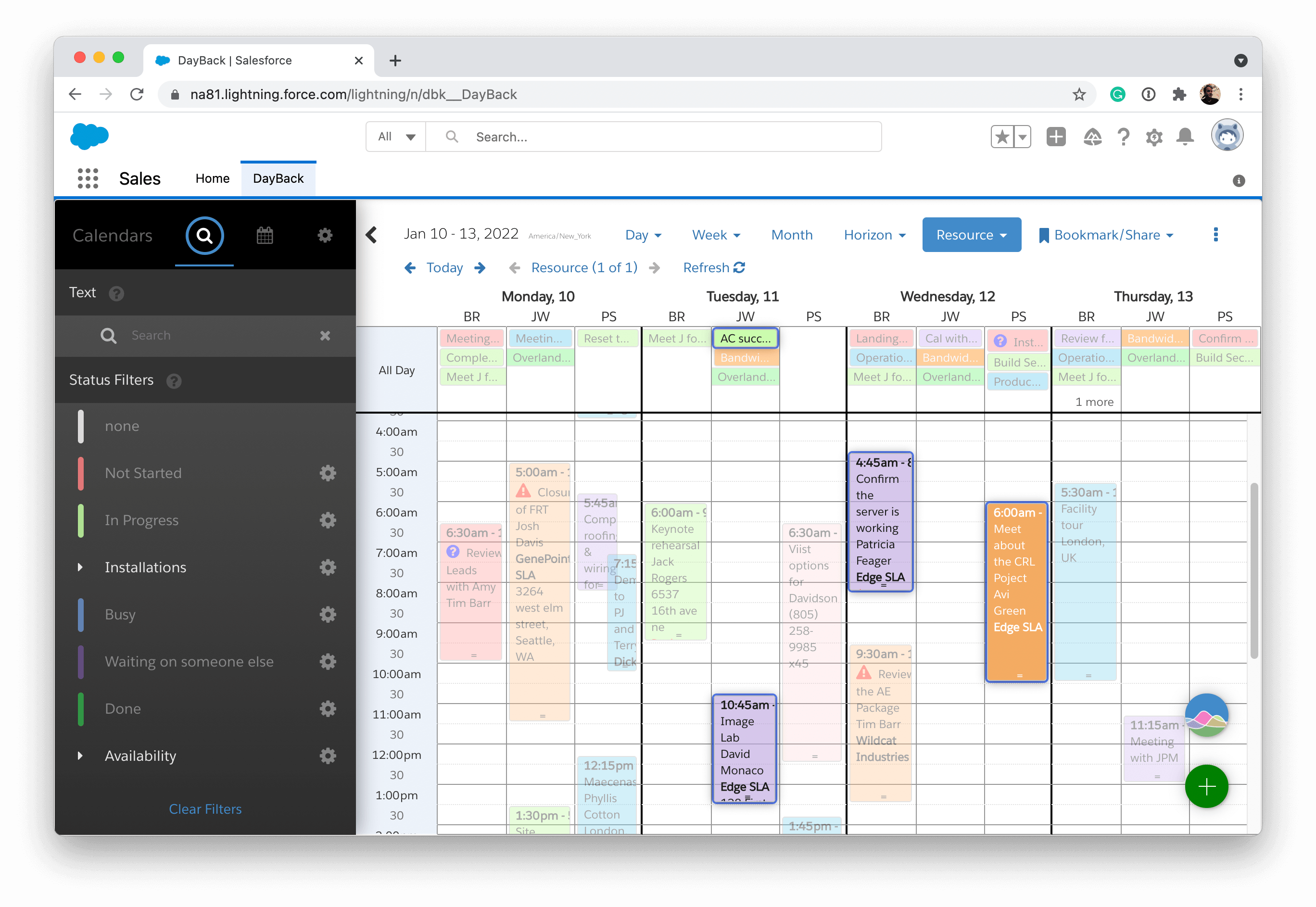
A full copy of this On Click Custom Event Action can be downloaded here.
Enjoy!
Leave a Reply